AI Marketing Chatbot: Build with Streamlit & OpenAI GPT-3.5-turbo with code source
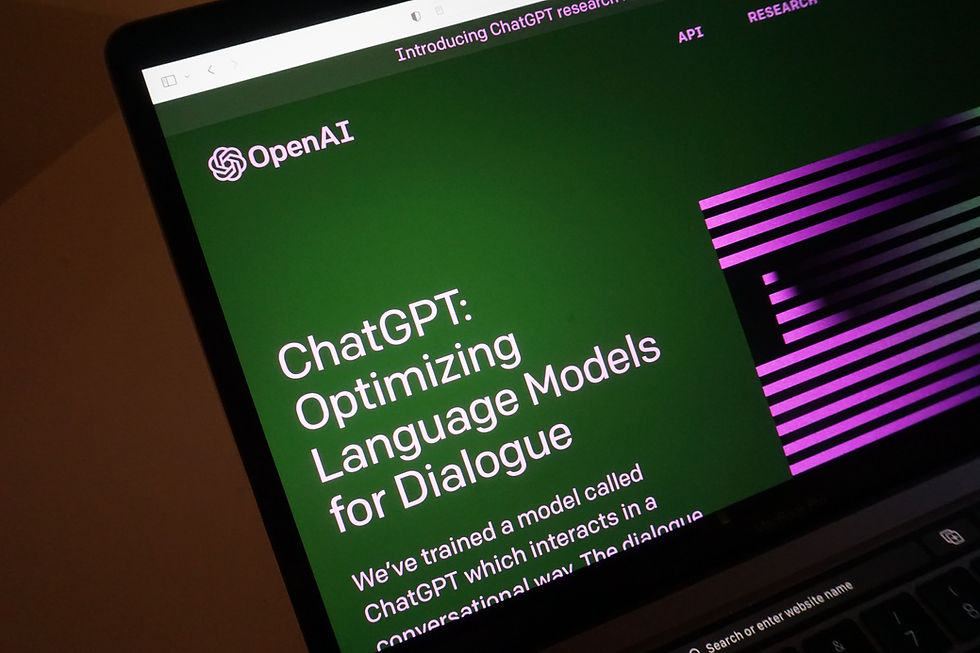
Table of Contents
1. Introduction📌:
Welcome to this tutorial! Today, we’re going to create an AI Marketing Chatbot. Imagine a virtual assistant that can answer all sorts of marketing questions for you, craft perfect outreach emails, generate persuasive sales scripts, and even help design your social media strategy - that’s what we’re building.
Here are some of the amazing things this chatbot can assist you with:
Crafting personalized and effective outreach emails that capture attention
Generating compelling sales scripts that resonate with your target audience
Offering insights on optimizing your marketing strategy
Suggesting improvements for your website’s SEO
Creating engaging content ideas for your social media platforms
We’ll use Python (a friendly and powerful programming language), Streamlit (a wonderful tool that turns your Python scripts into interactive web apps), and OpenAI’s super smart GPT-3.5-turbo model (the brain behind our chatbot that understands and generates human-like text). Plus, we’ll add a simple login system to keep it secure and exclusive to authorized users.
By the end of this tutorial, you'll have a smart, interactive assistant ready to boost your marketing efforts with data-driven advice and creative solutions.
So, are you excited? Let’s dive in! 🚀
2. What You Need Before Starting ✅:
First things first, let’s set up our toolbox. Here are the essential tools you need, and a bit about why we need each one:
Python 3.11:
This is our programming language, the foundation of our project. It’s popular, powerful, and great for beginners and experts alike.
Download it here.
Streamlit:
Think of this as our magic wand that turns our Python script into a beautiful and interactive web application. It’s super easy to use, and you’ll be amazed at what you can create with it.
Installation Command:
pip install streamlit
Get Streamlit here if you'd like to learn more.
OpenAI Python library:
This is the brain behind our chatbot. It connects our application to OpenAI’s advanced language models (like GPT-3.5-turbo), which are the systems that will understand and generate text for us.
Installation Command:
pip install openai
Check it out here for more details.
PIL (Python Imaging Library):
Sometimes, our chatbot will want to show images, and PIL is a library that helps us work with images in Python. It’s like our chatbot’s sketchbook.
Installation Command
pip install pillow
Download PIL here for more information.
Bonus: Virtual Environment (Highly Recommended)
Before you start installing these tools, I highly recommend setting up a virtual environment. This is like building a clean, isolated room where our project can live, away from all the noise and clutter of other projects. It ensures that the libraries and dependencies for different projects don't mix and clash.
Anaconda:
It’s a popular distribution of Python that makes it easy to manage virtual environments, and it comes with a lot of the tools data scientists and developers use every day pre-installed.
You can download Anaconda here.
3. Setting Up Your Project 👨💻
Create a new Python file (let’s call it app.py). In this file, we'll list all the tools we are going to use. It’s like gathering our ingredients before we start cooking:
import streamlit as st
import openai
import secret_key
import time
from PIL import Image
💡 Tip: secret_key here is a Python file where we'll store our sensitive information, like the OpenAI API key, so it’s not directly in our main script.
4. Making a Secure Login 🛡️
To keep our chatbot secure, we need two main things: a way to make sure only authorized users can access it, and a way to connect safely to OpenAI’s system. Here’s how we set that up:
Part 1: Setting Up User Authentication
First, we’ll create a simple list of usernames and passwords. This is like the guest list at an exclusive party—only people on the list can come in.
In Python, it looks like this (but please, make sure to use stronger passwords in a real-world application 😉):
users = {"admin": "password"}
💡 Tip: In a production environment, you should never store passwords in plain text like this. They should be hashed and salted using a library like bcrypt. This is just a simple example to get us started.
Part 2: Connecting to OpenAI’s System
To connect our chatbot to OpenAI’s system, we need a special code called an API key. Think of this as a secret handshake that lets OpenAI know our chatbot is a friend.
Here’s how we tell our Python script to use this key:
openai.api_key = secret_key.openai_api_key
In this code, secret_key.openai_api_key is a placeholder. You’ll replace this with your actual OpenAI API key, which you can get from the OpenAI website.
💡 Tip: Keep your API key secret, like a password. Never share it with anyone or publish it in publicly accessible code repositories. To make it more secure, you might want to use environment variables to store your API key, so it isn't directly in your script.
Part 3: Extra Security (Advanced, Optional)
For those who are familiar with web security, consider adding rate limiting (to prevent brute force attacks) and two-factor authentication for an extra layer of security.
This revised section breaks the step into parts, adds context and analogies for clearer understanding, highlights best practices with tips, and suggests advanced security options for those who are interested.
5. Making the Chatbot Talk 🗨️
Now, let's give our chatbot a voice! We want it to have engaging conversations with users. To do this, we'll write a function named communicate(). Think of this function as the chatbot's brain—it handles the entire back-and-forth dialog between the user and our chatbot.
Here’s a step-by-step breakdown of how this mini-script within our main script works:
def communicate():
try:
# 1. Retrieve the ongoing conversation
messages = st.session_state["messages"]
# 2. Get the user's message
user_message = {"role": "user", "content": st.session_state["user_input"]}
# 3. Add the user's message to the conversation
messages.append(user_message)
# 4. Send the conversation to OpenAI's model and get a response
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=messages
)
# 5. Extract the chatbot's reply from the response
bot_message = response["choices"][0]["message"]
# 6. Add the chatbot's reply to the conversation
messages.append(bot_message)
# 7. Clear the user input field for the next message
st.session_state["user_input"] = ""except Exception as e:
# Oops! If something goes wrong, this will catch the error and display it
st.write(f"Error: {e}")
Let’s delve into each step:
Retrieve the Ongoing Conversation: We want to keep track of the whole conversation, so we pick up where we left off. st.session_state["messages"] is like our chat history.
Get the User's Message: We gather what the user typed in. This is packed into a neat package (or "dictionary" in Python terms) with a role ("user") and the content of their message.
Add the User's Message to the Conversation: We then add this package to our ongoing conversation history.
Send the Conversation to OpenAI's Model and Get a Response: This is the magic step! We send the whole conversation to OpenAI, and its GPT-3.5-turbo model thinks deeply about it and crafts a reply for us.
Extract the Chatbot's Reply: OpenAI sends back a response, and we unpack it to get the message that our chatbot will send.
Add the Chatbot’s Reply to the Conversation: Just like we added the user's message to the conversation, we now add the chatbot’s reply.
Clear the User Input Field for the Next Message: After the chatbot responds, we clear the input field, making it nice and clean for the user’s next question or comment.
💡 Tip: Handling errors gracefully is crucial. The try...except structure here is like a safety net. If something unexpected happens, the chatbot will catch this and show an error message, rather than crashing.
6. Adding a Login Page 🔑
Let’s create a cozy little corner of our application: the Login Page. This page is the virtual front door of our chatbot, where users must enter their credentials to gain access. We’ll make a function called login() that displays this page. Think of this function as the chatbot’s friendly but firm security guard.
Here’s a step-by-step breakdown of how this security checkpoint works:
def login():
# 1. Display a Login section in the sidebar
st.sidebar.markdown("## Login")
st.sidebar.markdown("Please enter your credentials to login.")
# 2. Get the User's Input
username = st.sidebar.text_input("User Name")
password = st.sidebar.text_input("Password", type='password')
# 3. Check for Login Button Pressif st.sidebar.button("Login"):
# 4. Show a Progress Bar (for dramatic effect!)
progress_text = "Operation in progress. Please wait."
my_bar = st.sidebar.progress(0)
st.sidebar.text(progress_text)
for percent_complete in range(100):
time.sleep(0.01) # Optional, you might want to adjust this
my_bar.progress(percent_complete + 1)
# 5. Check the Credentialsif username in users and users[username] == password:
# 6. Successful Login!
st.session_state["user"] = username
st.success("Logged in successfully. Welcome back!")
else:
# 7. Failed Login
st.error("Incorrect username or password")
Let’s delve into each step:
Display a Login Section: We use the sidebar to create a designated area for login. It’s like the reception desk, asking “Who goes there?”
Get the User's Input: We provide fields for the user to type their username and password. The password field hides the input for security.
Check for Login Button Press: We wait for the user to press the “Login” button. Think of this as the user saying, “I’m ready. Check my ID.”
Show a Progress Bar (for dramatic effect!): As we check the credentials, we display a progress bar to let the user know something is happening behind the scenes. It’s like a drum roll before the big reveal.
Check the Credentials: Here’s where the serious security check happens. We compare the entered username and password with our list of authorized users.
Successful Login!: If the credentials match, we welcome the user into the app, like rolling out the red carpet.
Failed Login: If the credentials are incorrect, we display an error message. It’s our polite way of saying, “Sorry, you can’t come in.”
💡 Tip: In a real-world application, you should use secure methods to verify user credentials, possibly with a library to handle authentication and encryption for you.
7. Building the Main Chatbot Page 🤖
This is where we set up the main stage for our chatbot—the grand hall where all the magic happens! We are creating a function called main_app(), which is like the control center or the heart of our chatbot. It's where the user will spend most of their time, asking questions and receiving answers.
Here’s a step-by-step breakdown of how we set up this vibrant and interactive space:
def main_app():
# 1. Initialize the Conversationif "messages" not in st.session_state:
st.session_state["messages"] = [
{"role": "system", "content": system_prompt}
]
# 2. Set the Stage
st.title("Assistant AI Bot")
st.image("image.jpg")
st.write("🤖 Hello! Feel free to ask me anything.")
# 3. Sidebar for Marketing Insights
st.sidebar.markdown("## AUTOMATION MARKETING & OUTREACH")
st.sidebar.markdown("AI excellent marketing and sales expert. Expert on optimizing the conversion of marketing outreach and sales.")
# 4. Instructions for the User
st.markdown("**Instructions:**")
st.markdown("1. Type your message in the text input field below.")
st.markdown("2. Your message will be processed and an appropriate response will be displayed.")
# ... (continuation of the code)
Let’s delve into each step:
Initialize the Conversation: Before anything else, we make sure there’s a place in our app’s memory for the conversation to live. If the conversation hasn't started yet, we set up a welcoming message from the system.
Set the Stage: We add a title to our page, upload an image (maybe a cool chatbot avatar!), and write a greeting. This sets the tone for the interaction, like a friendly wave as the user walks in.
Sidebar for Marketing Insights: On the side, we have a special section dedicated to marketing and outreach. Think of this as a billboard highlighting the chatbot's skills in marketing and sales optimization. It's a way to showcase the special talents of our AI assistant.
Instructions for the User: To make sure our user knows how to interact with the chatbot, we lay out clear, step-by-step instructions. It’s like the guidebook for our virtual space, ensuring users know how to communicate with our AI friend.
💡 Tip: Consider adding more interactive elements, like buttons or sliders, that let the user customize their experience. For example, they might set the tone of the conversation (formal, casual), or select a specific topic they are interested in (SEO, email marketing, social media).
8. Running the Chatbot: The Grand Unveiling 🎉
Once you’ve written all the code, it’s time for the grand unveiling—it's like the opening night for a play that you’ve been preparing. This is the moment we bring our chatbot to life and let it start conversing with the world!
Here’s a step-by-step guide to launching your AI Marketing Chatbot:
Step 1: Open the Command Terminal 🖥️
Windows: Search for ‘cmd’ in the Start menu.
Mac: Search for ‘Terminal’ in Spotlight.
Linux: It’s usually found under ‘Accessories’ in your Applications menu.
This command terminal is like the backstage area of a theatre, where we pull all the strings.
Step 2: Navigate to Your Project Folder 🗂️
In the command terminal, navigate to the folder where your app.py file is saved using the cd (change directory) command. It’s like walking into the control room of our chatbot’s stage.
Example:
cd path/to/your/project/folder
Step 3: Run the Streamlit Command 🚀
Now, type the following command and press Enter:
streamlit run app.py
This command is the magic spell that tells Streamlit to run your Python file and start the chatbot. It’s like telling the actors, “Places, everyone! The show is starting!”
Step 4: Enjoy the Show 🎭
A new window will pop up in your web browser showing your AI Marketing Chatbot in action! It’s like the curtain rising on your play—everything you’ve worked on is now live and ready to perform.
💡 Tip: If the browser doesn't open automatically, the command terminal will usually display a URL (something like http://localhost:8501). Copy this URL and paste it into your web browser.
9. Trying it Out: Your Chatbot, Your Marketing Guru 🎙️
Ready for a chat? Now’s the time to put your AI Marketing Chatbot to the test. Imagine you’ve just hired a top-notch marketing consultant who is available 24/7, and this chatbot is your consultant. Let's explore its knowledge and creativity together!
Here’s a suggested workflow for getting started and some sample questions you might consider asking:
Step 1: Type Your Inquiry 🖋️
In the text input field (you’ll find this on your chatbot's main page), type a question or a request as you would to a marketing expert.
Try asking something like:
"Tell me about the benefits of AI in marketing?"
"Create an email outreach to offer web design services?"
"How can I optimize my sales funnel using AI?"
"What are the latest trends in automation marketing?"
Step 2: Press Enter or Click Send ⏎
After you’ve crafted your question, simply press the Enter key or click the 'Send' button. This is like saying, "Over to you!" to your chatbot.
Step 3: Absorb the Chatbot’s Wisdom 📖
Wait for a moment, and like a seasoned consultant, your chatbot will present its advice, strategies, or insights. Take time to read and understand the response—it's your personalized advice!
Step 4: Engage and Explore 💬
Don’t stop at one question. Engage with the chatbot as you would in a real conversation with a mentor. Ask follow-up questions, seek more details, or venture into a new marketing topic.
For instance:
"Can you provide examples of AI tools for social media?"
"How should I follow up with clients who don’t respond to my initial email?"
"What are some key metrics to track in a marketing campaign?"
💡 Tip 1: The chatbot is like a skilled colleague—the more specific and clear your questions, the more precise and actionable its advice will be.
💡 Tip 2: Challenge the chatbot creatively! Ask it to draft a catchy headline for your next blog post or a compelling call-to-action for a new landing page. It’s your on-demand creative department.
💡 Tip 3: If the chatbot's response isn’t quite what you need, rephrase your question or specify your request further. It’s all about fine-tuning the communication.
Step 5: Demo Video 🎥
For a hands-on guided tour of the chatbot, check out this demo video! It walks you through a variety of example questions, shows you how to interact with the chatbot, and highlights its capabilities—it’s like having a seasoned marketer right by your side, explaining the ropes.
10. Conclusion: A New Horizon in Marketing Assistance 🚀
Bravo! You've not only built an AI Marketing Chatbot but have also opened up a universe of possibilities. Your chatbot is more than just lines of code; it's a digital assistant, a marketing consultant, and a testament to the power of AI.
🌟 What You've Achieved:
Conversations with Flair: Your chatbot isn't just a bot; it's a conversationalist. It can handle intricate queries about marketing and provide insights on the fly.
Security First: With the login system you've integrated, you've ensured that the conversations remain exclusive to authorized users. It's a fortress guarding your digital realm.
Foundation for More: You've laid down a robust foundation. From here, scaling up or adding intricate features becomes a streamlined process.
🚀 What's Next?:
Expansion & Refinement: Think of new features, like integrating more data sources, expanding its knowledge base, or even giving it a voice!
Design Overhaul: While function is key, form is the charm. Consider giving your chatbot a UI/UX makeover to enhance user experience.
Learning Never Stops: AI thrives on data. The more it interacts, the better it can become. Continuous training can make it even more sophisticated.
Remember, this is just the beginning. Your chatbot is like a garden; with regular care, updates, and new features, it can bloom into something even more spectacular. The sky isn't the limit; it's just the beginning. Dive deep, explore, and let your creativity take flight.
📚 Resources & Further Exploration:
For those eager to dive deeper into the code, understand every nuance, or even contribute, here's the treasure trove: Full Code Repository on GitHub. It's a dynamic space, open for enhancements, optimizations, and community contributions.
Comments